ChatGPT has become the topic of discussion in many development circles, if you didn’t already know about it, ChatGPT is an AI-powered language model developed by OpenAI, capable of generating human-like text.
In this article, I’d show you how to use the OpenAI API with Java, and Spring Boot to correct and edit grammatical errors.
If you would like to learn more about external API integrations, read here and if you are new to Spring Boot, read here.
WHAT YOU WILL BUILD
A Web Application that converts ungrammatical statements into standard English.
WHAT YOU NEED
- JDK 1.8 and above
- Maven
- An IDE; using IntelliJ IDEA in this article
GETTing started
To get started, you need to sign up with OpenAI or login to generate your API key, this is necessary because the OpenAI API uses API keys for authentication.
Once you have your API key, you can start making requests to the API.
Building the application
There are different APIs in the documentation and they can be used for different use cases. Some examples are Chat Completion API, Image generation API, and Audio translation API.
Depending on what use case you have in mind, there are also different models.
However, as mentioned earlier, this integration is for grammar correction so we’d be making use of the Chat Completion API.
On the documentation, you’d find different libraries written in different languages but most of the examples are in Node and Python, it was a little hard connecting with Java, hence this article.
There’s an OpenAI library written in Java available, you can find it here. To connect to the library, I added this to my pom.xml:
<dependency>
<groupId>com.theokanning.openai-gpt3-java</groupId>
<artifactId>service</artifactId>
<version>0.14.0</version>
</dependency>
Request
For the grammar correction feature, the API/Object we would be using is the Chat Completion API.
The request body consists of different fields, most of which are optional, however, for this example, we would be working with the model and messages fields which are the only compulsory fields.
Model: there are different models that are compatible with our API, you can read more here, however, I would be using ‘gpt-3.5-turbo’, it’s cheaper and one of the most common.
Messages: the message field is an array of message objects, where each object has a role (either “system”, “user”, or “assistant”) and content.
The system message is what I used in formatting the request i.e. to specify what I wanted the system to do while the user message is the input text from the user, and the assistant message is the response from the system.
For the Request DTO, I only need to get the input text from the user as seen below:
public record GrammarCorrectionRequest(
String text
) {
}
Implementation service
Here’s where the business logic happens. Here, I integrate with the Chat Completion API using the java library mentioned earlier.
For the third line where you are required to pass a token, pass the API Key generated earlier.
P.S Pay attention to the System message to see where I set the behaviour of the assistant.
public ResponseEntity<GrammarCorrectionResponse> returnCorrectGrammar(GrammarCorrectionRequest request){
OpenAiService service = new OpenAiService(token);
ChatMessage systemMessage = new ChatMessage("system","You will be provided with statements, and your task is to convert them to standard English");
ChatMessage userMessage = new ChatMessage("user",request.text());
List<ChatMessage> messageList = new ArrayList<>();
messageList.add(systemMessage);
messageList.add(userMessage);
ChatCompletionRequest chatCompletionRequest = ChatCompletionRequest.builder()
.model("gpt-3.5-turbo")
.messages(messageList)
.build();
String correctText = service.createChatCompletion(chatCompletionRequest).getChoices().get(0).getMessage().getContent();
GrammarCorrectionResponse response = new GrammarCorrectionResponse();
response.setCorrectText(correctText);
return new ResponseEntity<GrammarCorrectionResponse>(response, HttpStatus.CREATED);
response
A number of fields get returned after the call is made successfully but the field we need is the message field which consists of role and content, the content is what contains the grammatically correct text and that is what I return to the user.
public class GrammarCorrectionResponse {
private String correctText;
public String getCorrectText() {
return correctText;
}
public void setCorrectText(String correctText) {
this.correctText = correctText;
}
}
Controller
Here, we have just one request; a POST request. The user sends a text as input and gets the grammatically correct text as output.
@RestController
@RequestMapping("/v1")
public class CorrectionController {
@Autowired
GrammarCorrectionService grammarCorrectionService;
@PostMapping
public ResponseEntity<GrammarCorrectionResponse> returnCorrectText(@RequestBody GrammarCorrectionRequest request){
return grammarCorrectionService.returnCorrectGrammar(request);
}
}
TESTING THE APPLICATION
I used postman to test the application. By default, your application starts on port 8080 but I configured mine to start on 8084.
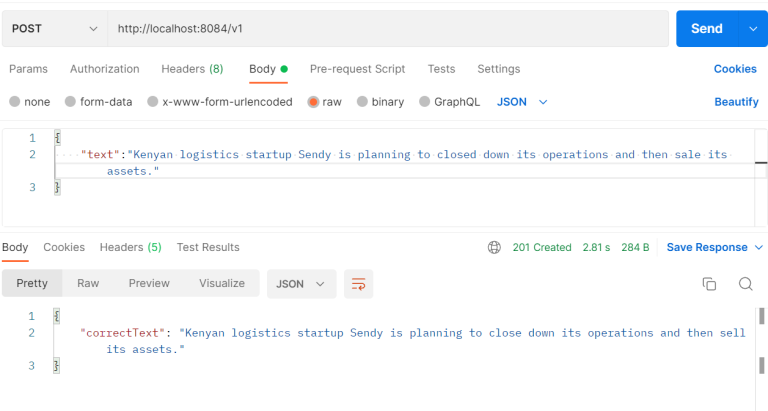
One error you might get from the test has to do with your API key so if you see the below error when testing, it’s because your free account quota has expired and you now need to pay to be able to carry out more tests.
{
“error”: {
“message”: “You exceeded your current quota, please check your plan and billing details.”,
“type”: “insufficient_quota”,
“param”: null,
“code”: “insufficient_quota”
}
}
Summary
As mentioned at the beginning of the article, there are many things you can do using the OpenAI API, however, I covered just one of them.
You can play around with other examples using the same method mentioned in the article.
I hope this was helpful. Leave me a comment or a question if you need further clarity. Cheers!